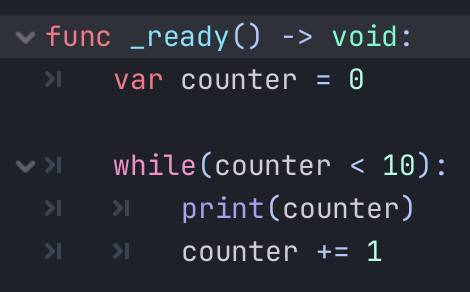
One essential concept when programming and developing games is the concept of a loop. Repeating functionality in your game will come up time and time again which is why all computer science and programming courses cover this concept.
In this guide we will cover what is a loop in Godot and how to use them in GDScript!
Prerequisites
This guide is designed for complete beginners to programming and Godot.
To follow this guide we recommend you at least first understand the basics of variables in Godot.
We recommend having the official Godot documentation available on the side for further details.
What is a Loop
A loop in all computer programming languages allows you to repeat steps of your code in a controlled way.
This prevents repeating parts of your code keeping your scripts clean and easy to read.
For Game Development, the best example of this would be the following. We have 4 enemies that we want to run the code for them to move on the screen. The naive approach would be to run the movement function for each of the enemies in your game.
Enemy1.Move()
Enemy2.Move()
Enemy3.Move()
Enemy4.Move()
This might work for 4 enemies, but when you have 100 enemies this way of programming becomes slow, tedious, and most importantly redundant.
Staying with this example, using loops we can run all of the enemy move functions with only a few lines of code!
Before we learn how to do this ourselves, we must understand the different types of loops available in the Godot Engine.
Types of Loops in Godot
While Loop
The while loop is the simplest loop. It will loop while the condition is true.
This condition must change back to false inside of the loop otherwise you have an infinite loop which freezes your game.
In GDScript, a while loop looks like this.
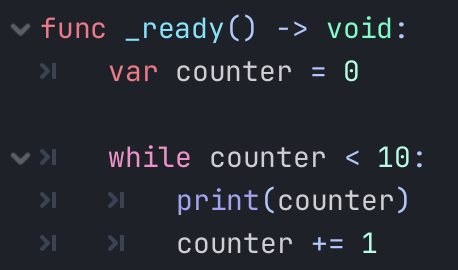
In this example the value “counter” is set to 0. We then start a while loop and our condition is that the loop runs while counter is less than 10.
Inside of the loop we print out the value of “counter” then add 1 to the counter.
Over the lifetime of the while loop this prints out 0 to 9 then finishes as the condition is met, counter is no longer less than 10!
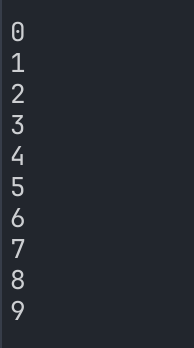
For Loop
The for loop is the most useful loop. It will loop only a specific amount of times. This can also be used to loop over all of the objects inside an array or all of the keys inside a dictionary!
Using the for loop in this way makes it the most common and useful loop. Storing your nodes and scenes inside of an array or dictionary can enable efficient and clean GDScripts when managing and using them.
Basic For Loop
In GDScript a simple for loop using the range function looks like this.
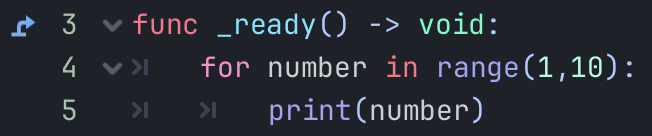
In this example the for loop runs from the range of 1 to 10. The range function gives us an array of numbers between 1 and 10 which we can loop for each item in the array.
The variable number is updated each time the loop runs. This means the first time it will be 1, the next would be 2, and so on until it reaches the end of the range, 10 in this example.
We then print the value of the variable number to the output.
After running this code the output looks like this.
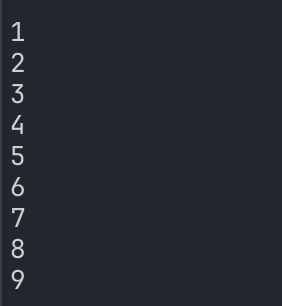
For Loop using an Array
In GDScript a for loop using an array looks like this.
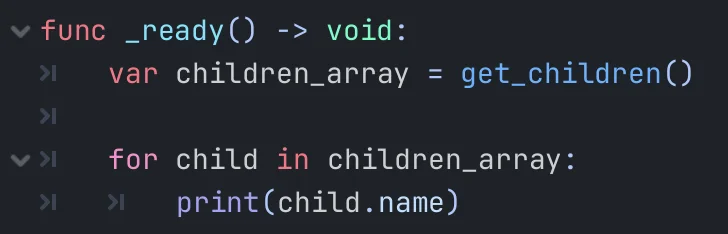
In this example we get all of the child nodes our current node has.
Then we use a for loop and use the keyword in. This mean child is the node inside of children_array. Each time the for loop runs, child represents the next item in the array.
Inside the for loop, now we print the name value of the child.
Using a for loop in this way allows us to only write the code once keeping the interaction with our array clean and simple.
After running this code the output looks like this.
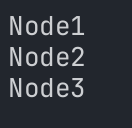
For Loop using a Dictionary
And finally in GDScript a for loop using a dictionary looks like this.
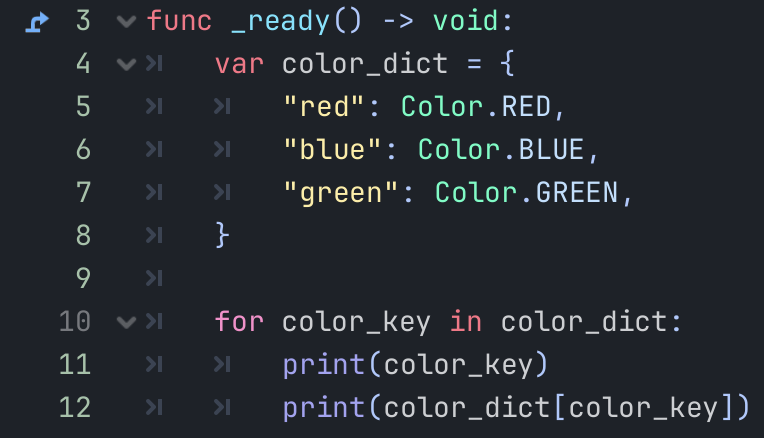
In this example we have a dictionary containing the names of the colors as the dictionary key and the color RGBA value as the dictionary value.
Each time the for loop runs color_key is the key and not the value of the dictionary. This is then used in the for loop by printing the key and then the value by accessing the dictionary with the square brackets.
After running this code the output looks like this.
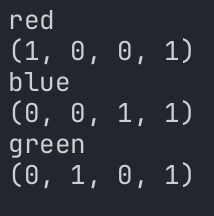
Statements
Statements are words you can use inside of your loops to affect the flow.
Break
Using the word “break” inside of your loop will exit the current loop scope. This completely stops the loop and continues the rest of your code.
This is especially useful inside of while loops that are infinite.
In this example if number equals 5, the loop will break. This means it will only run from 1 to 5 instead of the full 1 to 10.
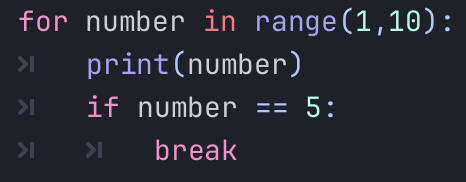
Continue
Using the word “continue” inside of your for loop will end the current iteration of the loop and run the next item in the loop. This does not fully exit the loop like break!
For example, if you want the loop to stop at a specific point, you simply put continue!
In the example below we use continue to skip the print when the counter is less than or equal to 5!
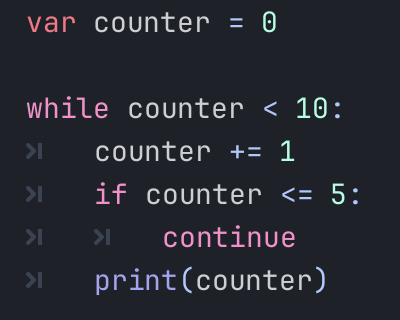
Conclusion
By following this guide you now understand what is a loop in Godot!
You will use these loops in almost every single script you make for your project so we recommend learning further by reading these resources on loops:
Leave a Reply