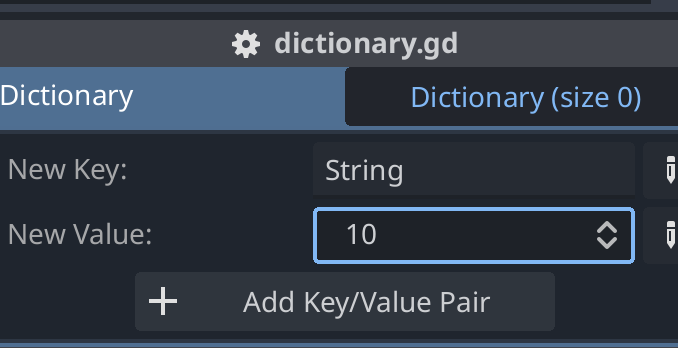
When storing data that needs to be easily retrieved, the Dictionary is the best and easiest tool for the job in any Godot developers arsenal.
In this guide we explain the basics of what a dictionary is, how to create one, retrieving the data, and how to manage your dictionary all in GDScript inside of the Godot Engine!
Prerequisites
Before you start this guide, we recommend you understand the basics of the Godot Editor and also how to use Variables in the Godot Engine, specifically GDScript.
Click here to read our guide on variables in Godot.
What is an Dictionary
The Dictionary, (also known in other programming languages as a Map) is a data structure that allows you to store data along side a key.
This data is tied to the key and allows easy storage and access of the data without any complex code or slow find functions.
The most important aspect to understand of the Dictionary is the Key and Value.
- The Key is used to locate the information. This must be unique!
- The Value can be any data you want associated to the key.
For example, lets say we want to store and retrieve all of the items based on a specific “rarity” words such as “common”, “rare”, or “ultra rare”.
We add all of our items as the value into the Dictionary using these rarity words as the key:
- Common – Dirt, Rocks, Wood
- Rare – Iron, Copper, Tin
- Ultra Rare – Diamond, Platinum, Gold
We then ask the dictionary to give us all the value associated with the key. One example would be to ask the dictionary for all of the “rare” items.
This returns us the list of rare items: iron, copper, and tin.
This takes no special code and is very easy to use any script that needs easy storage and retrieval of data in your Godot game project!
How to use an Dictionary in Godot
Declaring an Dictionary
The dictionary is a variable so it must be declared as usual. We declare the dictionary variable by simply making it equal curly brackets as shown in the image below.

Unlike arrays, in Godot 4.3 and below, you cannot declare dictionaries as statically typed. This means you have to make sure the data you are adding into your dictionary is correct for your use case.
However, like all other variables, we can export this to the editor to be managed using the @export keyword.

When your script is added to a node, you can see the exported dictionary, it’s size, and the options to add a new key value pair.
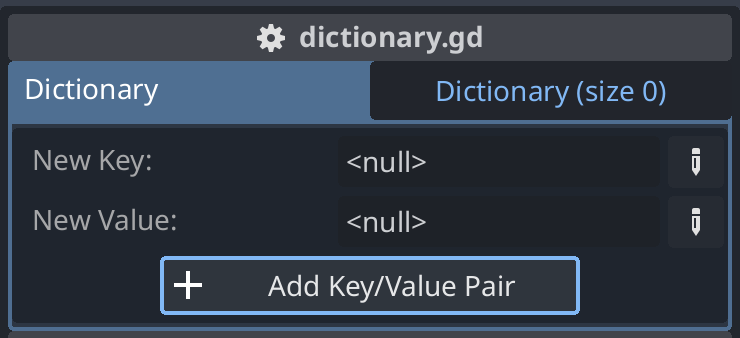
Clicking the small pencil icons next to New Key and New Value, we can see a large list of available types. When exporting dictionaries to the editor, you must tell it the specific types used.
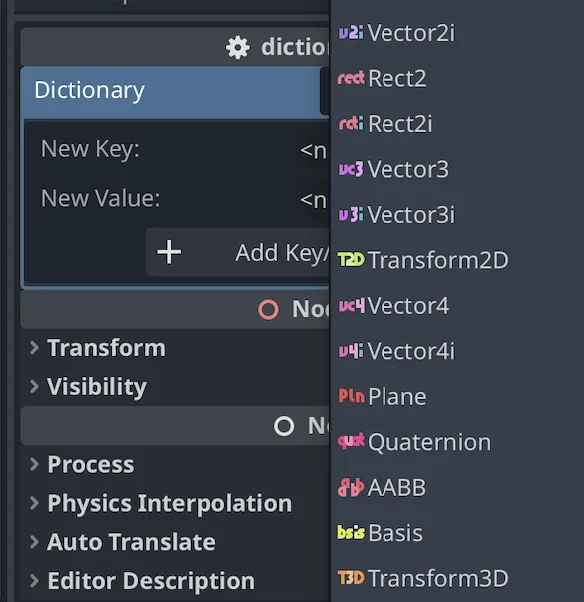
As shown in the image below, we selected the String type for our New Key and the int type for our New Value. We then press Add Key/Value Pair to add our key and value to the dictionary!
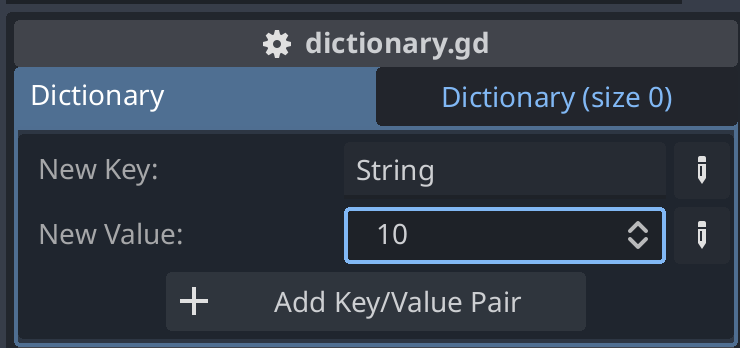
Adding items to a Dictionary
Adding a new element to a dictionary is as simple as providing a key and value!
Dictionaries are interacted with square brackets.
Any data you put inside of the square brackets is used as the key.
Using the equals on this then sets the value of that key.
As shown in the image below, we set the value 10 into the key string “key”.
Setting the value and adding it are the same. If it does not exist it will add the new key to the dictionary.
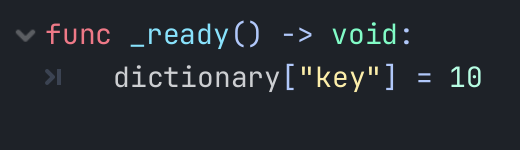
Retrieving items from a Dictionary
Retrieving items from the dictionary is even easier!
As shown in the image below, simply access the dictionary using the square brackets and put the key you want inside!
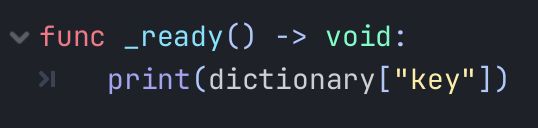
Note: If your dictionary does not have the key it will cause and error in your code!
Use the has function inside of an if statement to check if your key exists before using the value.
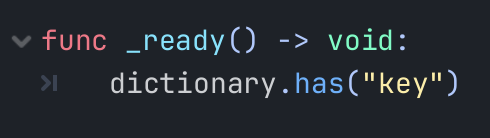
If we want to get all of the keys from our dictionary, simple use the keys function. This will give us an array that contains all of the keys which can be used in loops or other code.
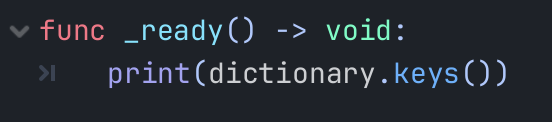
Similar to the keys function, we can use the values function to get all of the values contained in our dictionary as an array for looping or other uses.
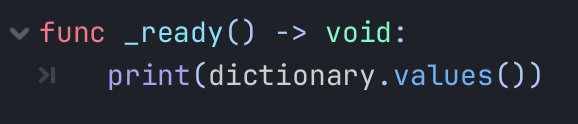
Managing a Dictionary
There are many useful functions to manage the keys and values inside your dictionary. Here are the most useful that you will use in your scripts.
The first is the clear function. This removes all keys and values from the dictionary.
This destroys all data inside of the dictionary. Be careful with this function!
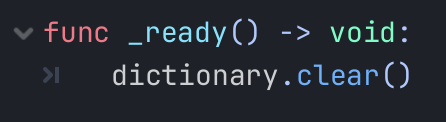
Next is the merge function. This is the same as the append array function if you are familiar with that from our last guide.
This merges two dictionaries together, adding our second dictionary to the first. It now contains the keys and values of both.
We simply use the merge function and pass our second dictionary in and our two dictionaries are merged.
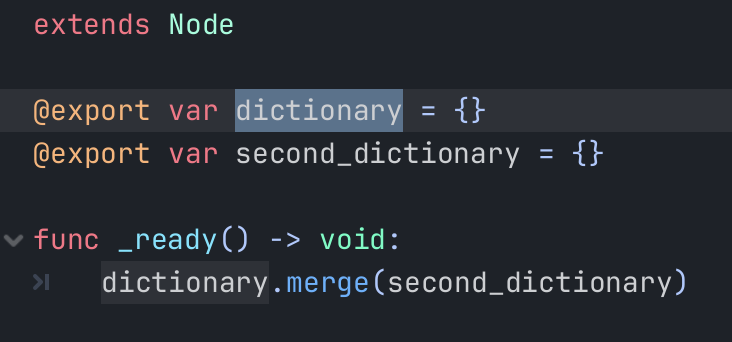
The last management function is the size function. This returns the amount of keys inside of our array!
If you have 5 keys in your array with data, the size function will return the integer 5.
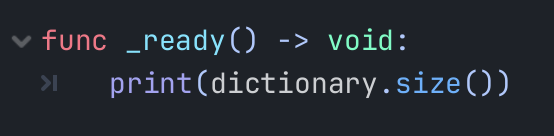
Advanced Usage
Now that you have the basics down, we encourage along your developer journey to learn how to use loops.
Loops allow you to go through each key and value in your dictionary and run code on them.
This is especially useful for looping through objects.
To learn how to use loops in Godot, click here to read our guide on loops in Godot!
Conclusion
Now you understand how to use Dictionaries in Godot!
Dictionaries are an essential tool when storing uniquely identifiable information and objects in your Godot project.
With this knowledge you can now tackle more complex topics that we cover on BlueRobotGuru!
Leave a Reply